
Because all digits are not whitespace, and all whitespace characters are not digits, matches any character digit, whitespace, or otherwise. The former, however, matches any character that is either not a digit, or is not whitespace. The latter matches any character that is neither a digit nor whitespace. īe careful when using the negated shorthands inside square brackets. \D is the same as, \W is short for and \S is the equivalent of. The above three shorthands also have negated versions. matches a hexadecimal digit, and is equivalent to if your flavor only matches ASCII characters with \d. When applied to 1 + 2 = 3, the former regex matches 2 (space two), while the latter matches 1 (one). matches a single character that is either whitespace or a digit. \s \d matches a whitespace character followed by a digit. Shorthand character classes can be used both inside and outside the square brackets. But JavaScript does match all Unicode whitespace with \s. In flavors that support Unicode, \s normally includes all characters from the Unicode “separator” category. Most flavors also include the vertical tab, with Perl (prior to version 5.18) and PCRE (prior to version 8.34) being notable exceptions. That is: \s matches a space, a tab, a carriage return, a line feed, or a form feed. In all flavors discussed in this tutorial, it includes. Again, which characters this actually includes, depends on the regex flavor. Again, Java, JavaScript, and PCRE match only ASCII characters with \w. XML Schema and XPath even include all symbols in \w. Connector punctuation other than the underscore and numeric symbols that aren’t digits may or may not be included. Letters and digits from alphabetic scripts and ideographs are generally included. There is a lot of inconsistency about which characters are actually included. In most flavors that support Unicode, \w includes many characters from other scripts. Notice the inclusion of the underscore and digits. These Unicode flavors match only ASCII digits with \d. Notable exceptions are Java, JavaScript, and PCRE. In most flavors that support Unicode, \d includes all digits from all scripts.
#Javascript does not equal shorthand code#
The ternary operator can be used to write shorter code for a simple if else statement.Since certain character classes are used often, a series of shorthand character classes are available. Using logical operators to test multiple conditions can replace nested if else statements. If you feel like the if else statement is long and complicated, then a switch statement could be an alternative option.
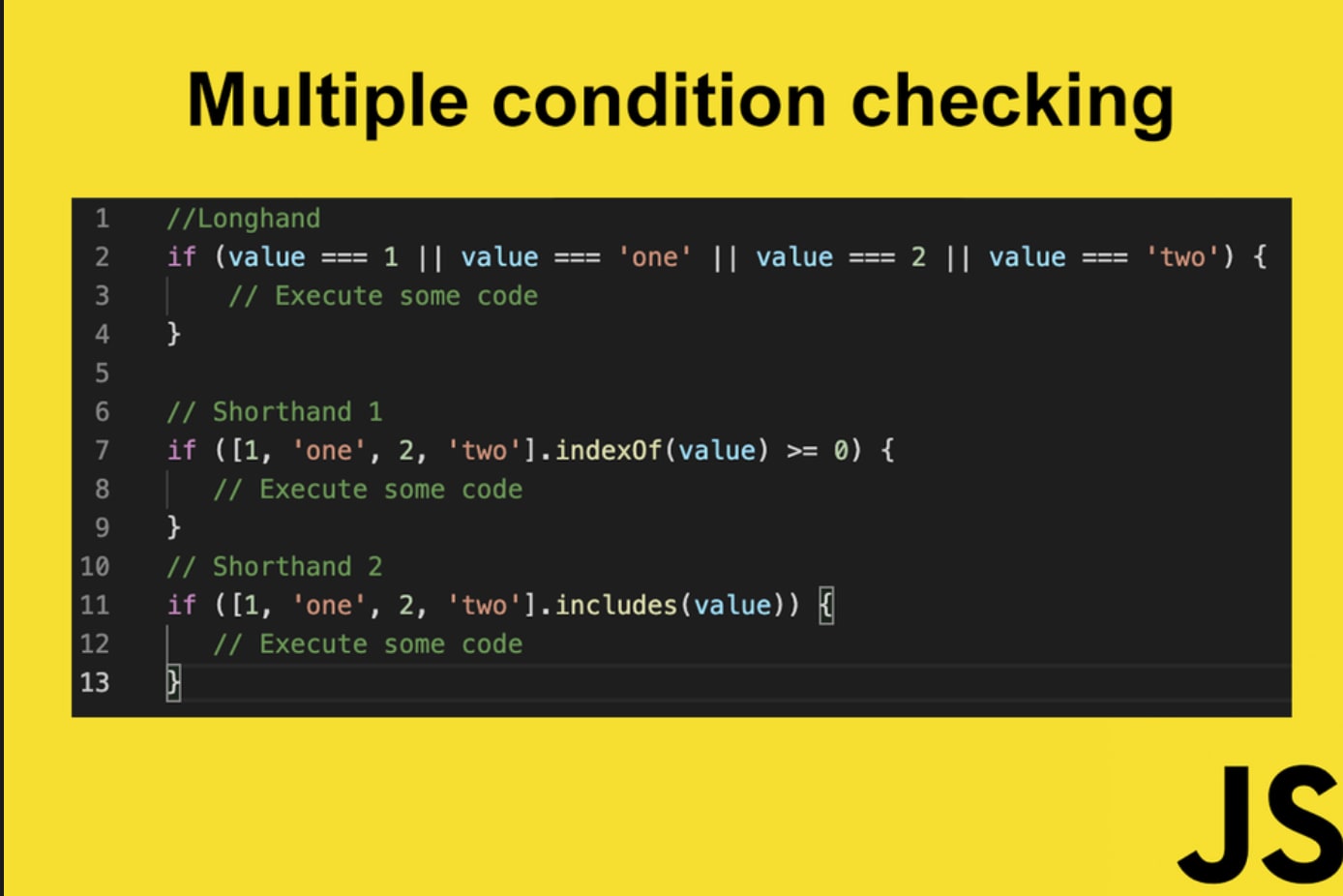
There will be times where you want to test multiple conditions and you can use an if.else if.else statement. If the condition is falsy, then the else block will be executed. If else statements will execute a block of code when the condition in the if statement is truthy. This is what the code would look like using an if else statement: const age = 32 const age = 32 Ĭonst citizen = age >= 18 ? "Can vote" : "Cannot vote" In this example, since age is greater than 18, then the message to the console would be "Can vote". If the condition is false, then the code after the : would execute. The condition goes before the ? mark and if it is true, then the code between the ? mark and : would execute.

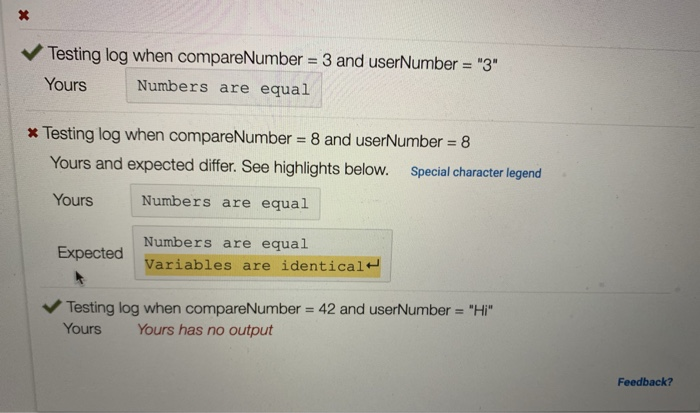
This is the basic syntax for a ternary operator: condition ? if condition is true : if condition is false The word ternary means something composed of three parts. If you have a short if else statement, then you might choose to go with the ternary operator. if (condition is true) Conditional (ternary) operator in JavaScript Truthy and falsy values are converted to true or false in if statements. The if.else is a type of conditional statement that will execute a block of code when the condition in the if statement is truthy. What is an if.else statement in JavaScript? We will also look at the conditional (ternary) operator which you can use as a shorthand for the if.else statement. In this article, I will explain what an if.else statement is and provide code examples. There will be times where you will want to write commands that handle different decisions in your code.įor example, if you are coding a bot, you can have it respond with different messages based on a set of commands it receives.
